* Ubuntu 18.04
* PHP7.2
* Laravel 7
* Vue 2.xx
* MariaDB
1. 패키지 설치
apt update
apt upgrade
# nginx 설치
apt-get install nginx
# php 설치
apt install php7.2-fpm php7.2-mysql php7.2-zip php7.2-gd php7.2-mbstring php7.2-xml mcrypt
# mariadb 설치
apt install mariadb-server php-mysql
2. php.ini 설정
# php.ini 파일을 vim 에디터로 open
vim /etc/php/7.2/fpm/php.ini
# cgi.fix_pathinfo를 0으로
cgi.fix_pathinfo=0
3. NGINX 설정
vim /etc/nginx/sites-available/default
server {
listen 80 default_server;
listen [::]:80 default_server;
root /webserver/laravel/public;
index index.php index.html index.htm;
server_name _;
location / {
try_files $uri $uri/ /index.php?$query_string;
}
location = /favicon.ico { access_log off; log_not_found off; }
location = /robots.txt { access_log off; log_not_found off; }
error_page 404 /index.php;
location ~ \.php$ {
fastcgi_pass unix:/var/run/php/php7.2-fpm.sock;
fastcgi_index index.php;
fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name;
include fastcgi_params;
}
}
4. MariaDB 설정 + 테스트 계정/데이터 추가
# 알아서 설정하시오..
mysql_secure_installation
mysql
# 테스트 DB 생성
CREATE DATABASE test DEFAULT CHARACTER SET utf8;
use test
# 테스트 테이블 생성 + 테스트 데이터 추가
CREATE TABLE mydata(number int NOT NULL, title varchar(100) NOT NULL, content text NOT NULL);
INSERT INTO mydata VALUES(1, "제목1", "내용1");
INSERT INTO mydata VALUES(2, "제목2", "내용2");
INSERT INTO mydata VALUES(3, "제목3", "내용3");
# 사용자 계정 생성 + 권한 추가
create user 'test_user'@'%' identified by '1234';
GRANT ALL privileges ON test.* to test_user@'%';
5. composer 설치
cd ~
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
HASH="$(wget -q -O - https://composer.github.io/installer.sig)"
php -r "if (hash_file('SHA384', 'composer-setup.php') === '$HASH') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;"
php composer-setup.php --install-dir=/usr/local/bin --filename=composer
#확인
composer
6. Laravel 설치
mkdir /webserver
cd /webserver
composer create-project laravel/laravel
cd laravel
composer install
chown -R :www-data .
chmod -R 775 ./storage/
service php7.2-fpm restart
service nginx restart
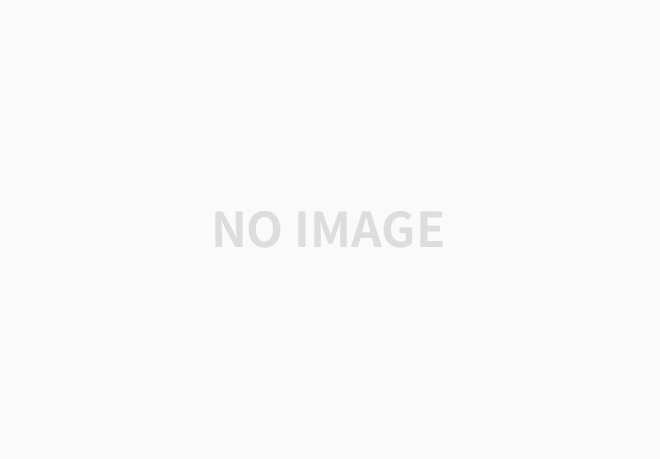
7. Vue 설치 + Laravel 연동
cd /webserver/laravel
sudo npm install
- npm 설치 안돼있을 때
apt-get install npm
sudo npm install
- 버전오류나면(npm ERR! argv "/usr/bin/node" "/usr/bin/npm" "install")
sudo npm cache clean --force
sudo npm install -g n
sudo n stable
sudo npm install -g npm
sudo npm install
이어서 설치
npm install vue
npm install vue-router
npm run watch
위까지 완료후 /webserver/laravel/package.json 파일에 아래 의존성이 제대로 들어갔는지 확인
"dependencies": {
"vue": "^2.6.14",
"vue-router": "^3.5.2"
}
8. vue 연동 blade 파일 생성
mkdir /webserver/laravel/resources/views/vue.blade.php
/* vue.blade.php */
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel</title>
</head>
<body>
<div id="app">
<vuemain></vuemain>
</div>
</body>
<script src="{{mix('/js/app.js')}}"></script>
</html>
9. Laravel Route 설정
/* /webserver/laravel/routes/web.php */
/* 아래코드 추가 */
Route::get('/app', function () {
return view('vue');
});
Route::any('/app/{slug}', function(){
return view('vue');
});
10. app.js 설정
require('./bootstrap');
window.Vue = require('vue')
import router from './router'
Vue.component('mainapp', require('../components/mainapp.vue').default)
const app = new Vue({
el:'#app',
router
})
11. 테스트 Vue 파일 + 디렉터리 설정 + Vue Router 설정
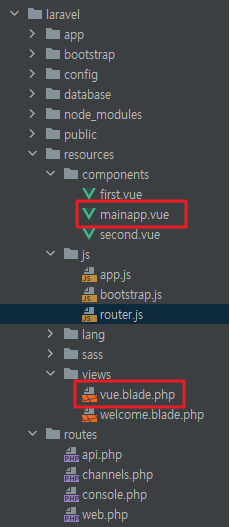
* vue.blade.php가 main_app.vue의 부모
* main_app.vue가 first.vue, second.vue들의 부모
- main_app.vue를 URL변경시에도 고정되며 내부적으로 first.vue, second.vue 컴포넌트들을 교체
router.js
/* touch /webserver/laravel/resources/js/router.js */
import Vue from 'vue';
import Router from 'vue-router';
Vue.use(Router);
import first from '../components/first'
import second from '../components/second'
const routes =[
{
name:'first',
path:'/app/first',
component:first
},
{
name:'second',
path:'/app/second',
component:second
}
]
export default new Router({
mode:'history',
routes
})
mainapp.vue
/* touch /webserver/laravel/resources/components/mainapp.vue */
<template>
<div>
<router-view />
</div>
</template>
first.vue
/* touch /webserver/laravel/resources/components/first.vue */
<template>
<div>first Page</div>
</template>
<script>
export default {
name: "first"
}
</script>
<style scoped>
</style>
second.vue
/* touch /webserver/laravel/resources/components/second.vue */
<template>
<div>second Page</div>
</template>
<script>
export default {
name: "second"
}
</script>
<style scoped>
</style>
결과
- URL : 도메인(IP)
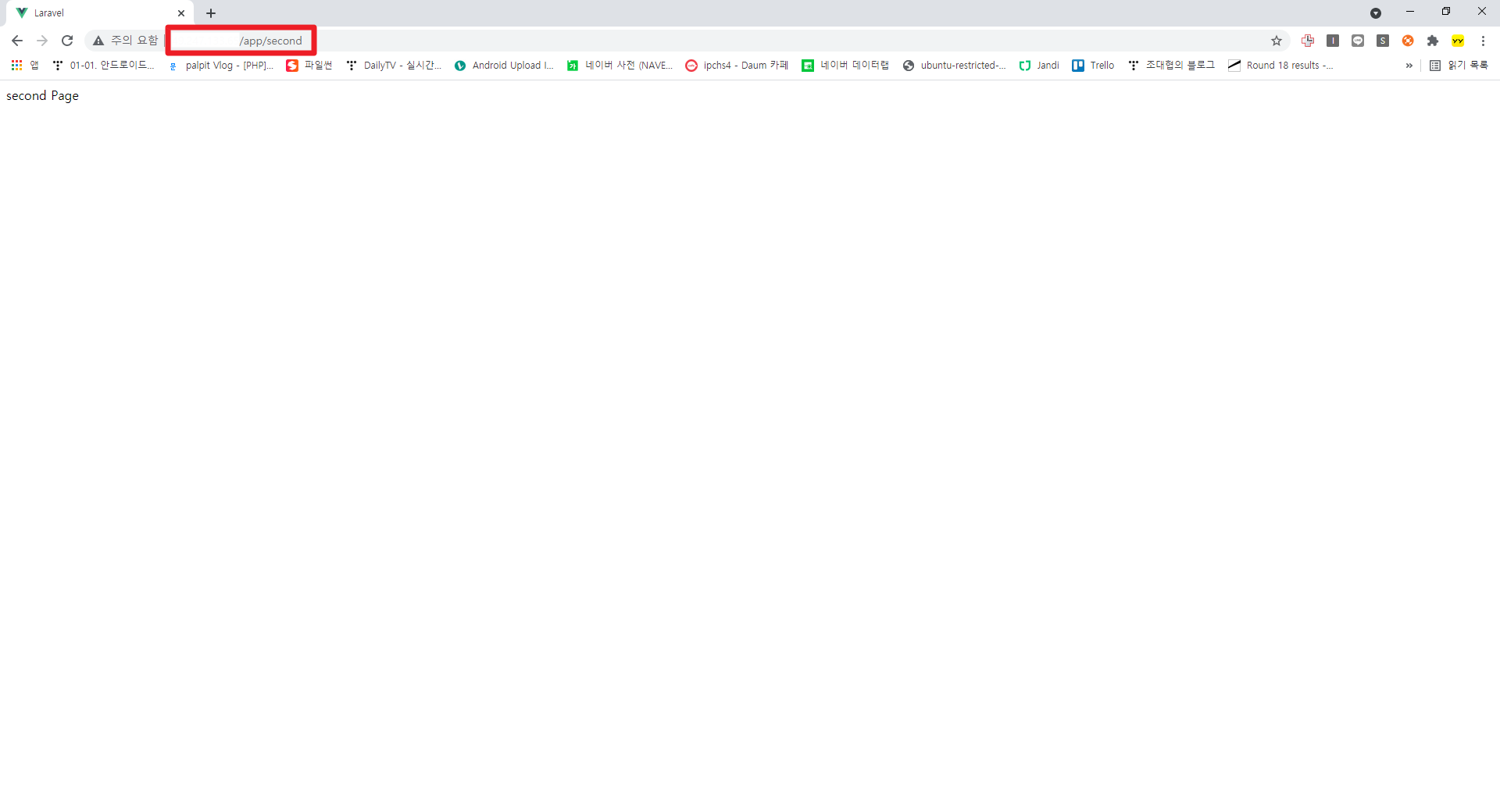
- URL : 도메인(IP)/app/first
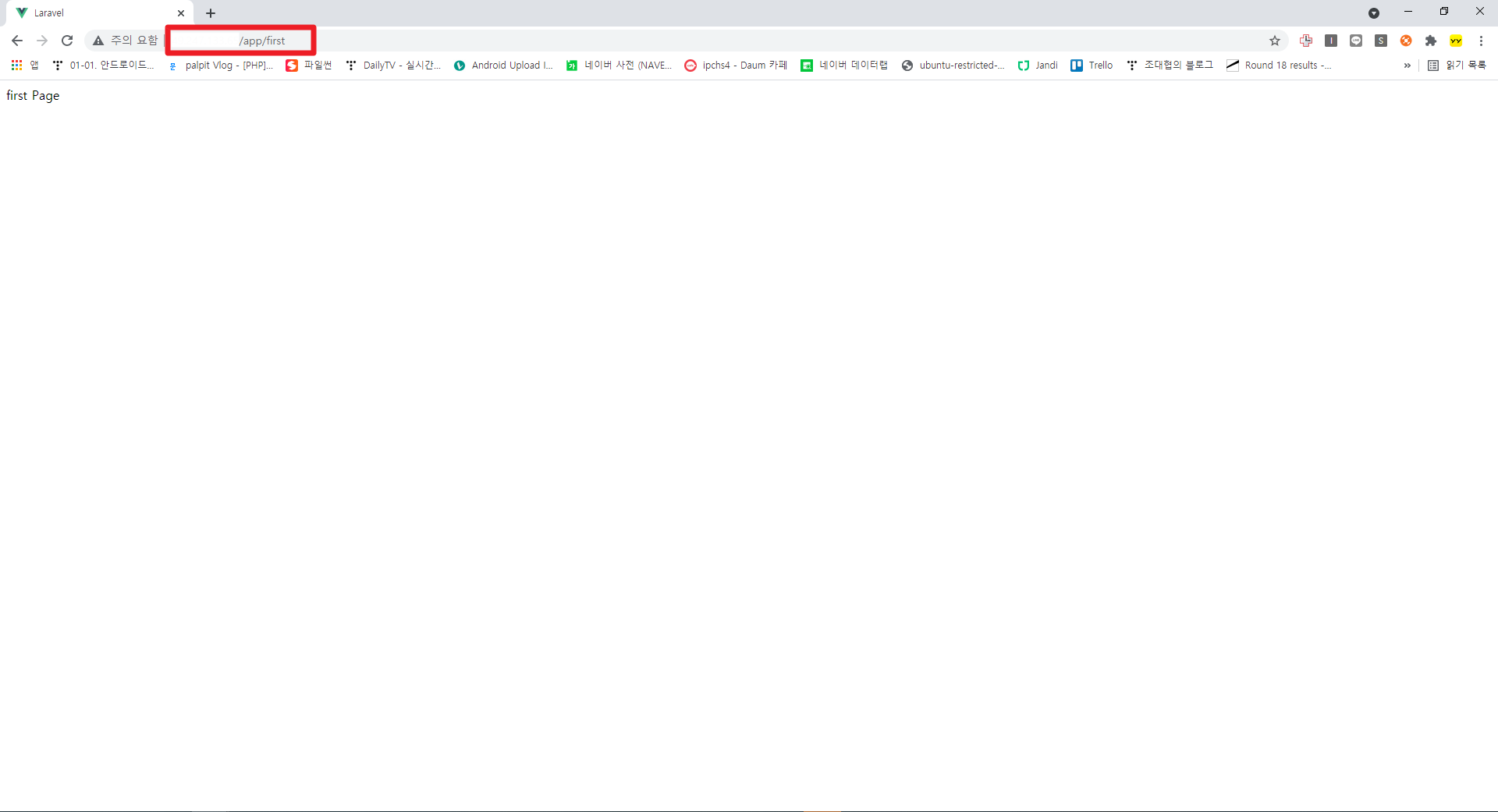
- URL : 도메인(IP)/app/second
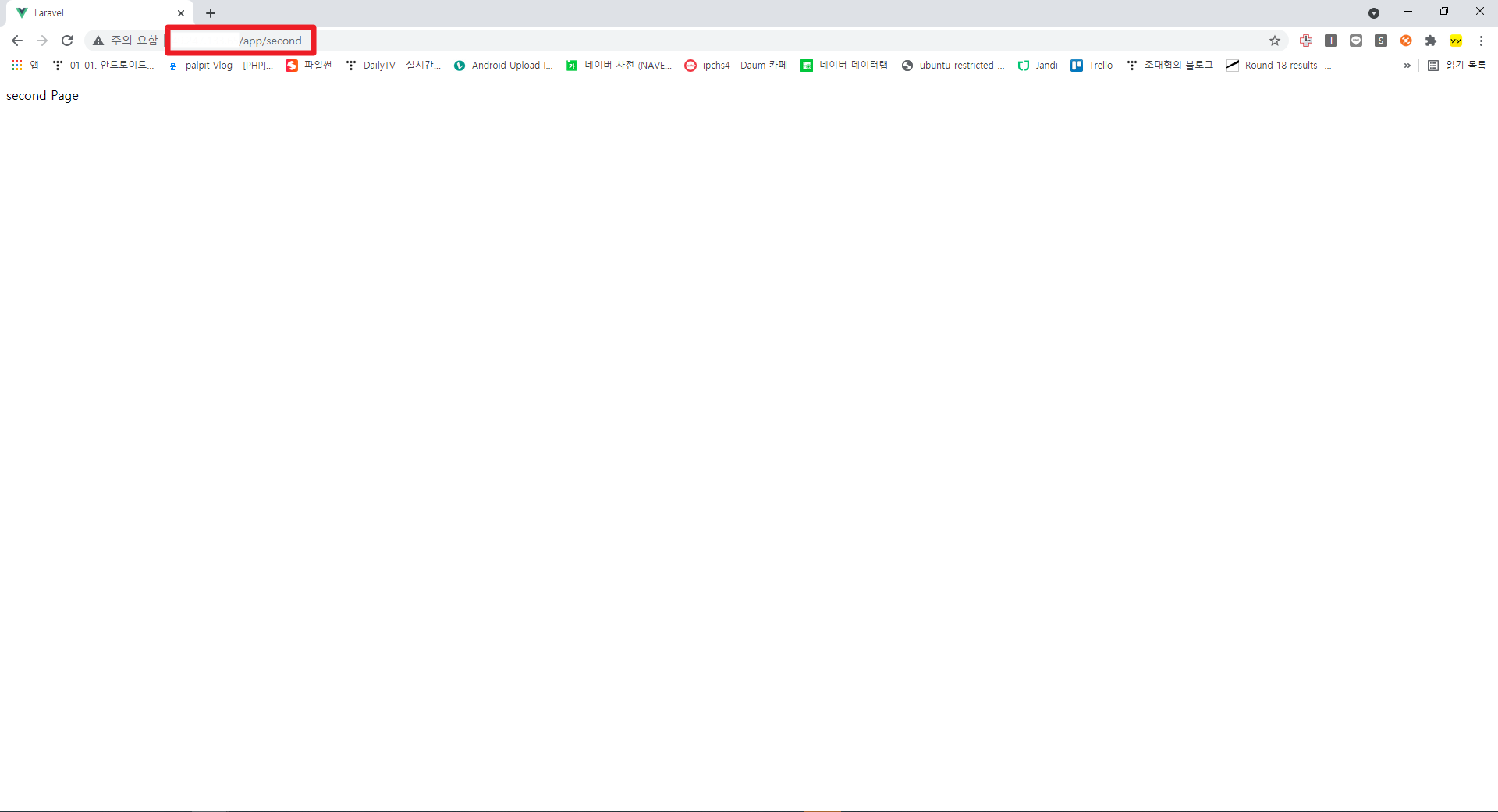
'PHP' 카테고리의 다른 글
[이카운트] ECOUNT API 연동(4) (2) | 2021.12.10 |
---|---|
[이카운트] ECOUNT API 연동(3) (0) | 2021.12.10 |
[이카운트] ECOUNT API 연동(2) (0) | 2021.12.10 |
[이카운트] ECOUNT API 연동(1) (0) | 2021.12.10 |
AMP 컴파일설치(PHP) (0) | 2019.03.31 |
댓글